mirror of
https://github.com/LemmyNet/lemmy.git
synced 2024-11-01 02:00:01 +00:00
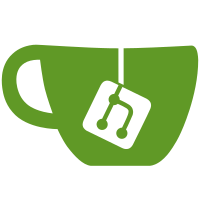
* Adding a way to GetComments for a community given its name only. * Adding getcomments to api docs. * A first pass at locally working isomorphic integration. * Testing out cargo-husky. * Testing a fail hook. * Revert "Testing a fail hook." This reverts commit0941cf1736
. * Moving server to top level, now that UI is gone. * Running cargo fmt using old way. * Adding nginx, fixing up docker-compose files, fixing docs. * Trying to re-add API tests. * Fixing prod dockerfile. * Redoing nightly fmt * Trying to fix private message api test. * Adding CommunityJoin, PostJoin instead of joins from GetComments, etc. - Fixes #1122 * Fixing fmt. * Fixing up docs. * Removing translations. * Adding apps / clients to readme. * Fixing main image. * Using new lemmy-isomorphic-ui with better javascript disabled. * Try to fix image uploads in federation test * Revert "Try to fix image uploads in federation test" This reverts commita2ddf2a90b
. * Fix post url federation * Adding some more tests, some still broken. * Don't need gitattributes anymore. * Update local federation test setup * Fixing tests. * Fixing travis build. * Fixing travis build, again. * Changing lemmy-isomorphic-ui to lemmy-ui * Error in travis build again. Co-authored-by: Felix Ableitner <me@nutomic.com>
65 lines
1.5 KiB
Rust
65 lines
1.5 KiB
Rust
use crate::{
|
|
schema::{category, category::dsl::*},
|
|
Crud,
|
|
};
|
|
use diesel::{dsl::*, result::Error, *};
|
|
use serde::Serialize;
|
|
|
|
#[derive(Queryable, Identifiable, PartialEq, Debug, Serialize)]
|
|
#[table_name = "category"]
|
|
pub struct Category {
|
|
pub id: i32,
|
|
pub name: String,
|
|
}
|
|
|
|
#[derive(Insertable, AsChangeset)]
|
|
#[table_name = "category"]
|
|
pub struct CategoryForm {
|
|
pub name: String,
|
|
}
|
|
|
|
impl Crud<CategoryForm> for Category {
|
|
fn read(conn: &PgConnection, category_id: i32) -> Result<Self, Error> {
|
|
category.find(category_id).first::<Self>(conn)
|
|
}
|
|
|
|
fn create(conn: &PgConnection, new_category: &CategoryForm) -> Result<Self, Error> {
|
|
insert_into(category)
|
|
.values(new_category)
|
|
.get_result::<Self>(conn)
|
|
}
|
|
|
|
fn update(
|
|
conn: &PgConnection,
|
|
category_id: i32,
|
|
new_category: &CategoryForm,
|
|
) -> Result<Self, Error> {
|
|
diesel::update(category.find(category_id))
|
|
.set(new_category)
|
|
.get_result::<Self>(conn)
|
|
}
|
|
}
|
|
|
|
impl Category {
|
|
pub fn list_all(conn: &PgConnection) -> Result<Vec<Self>, Error> {
|
|
category.load::<Self>(conn)
|
|
}
|
|
}
|
|
|
|
#[cfg(test)]
|
|
mod tests {
|
|
use crate::{category::Category, tests::establish_unpooled_connection};
|
|
|
|
#[test]
|
|
fn test_crud() {
|
|
let conn = establish_unpooled_connection();
|
|
|
|
let categories = Category::list_all(&conn).unwrap();
|
|
let expected_first_category = Category {
|
|
id: 1,
|
|
name: "Discussion".into(),
|
|
};
|
|
|
|
assert_eq!(expected_first_category, categories[0]);
|
|
}
|
|
}
|