mirror of
https://github.com/LemmyNet/lemmy.git
synced 2024-11-10 22:45:02 +00:00
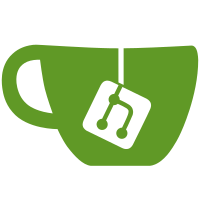
* Adding a way to GetComments for a community given its name only. * Adding getcomments to api docs. * A first pass at locally working isomorphic integration. * Testing out cargo-husky. * Testing a fail hook. * Revert "Testing a fail hook." This reverts commit0941cf1736
. * Moving server to top level, now that UI is gone. * Running cargo fmt using old way. * Adding nginx, fixing up docker-compose files, fixing docs. * Trying to re-add API tests. * Fixing prod dockerfile. * Redoing nightly fmt * Trying to fix private message api test. * Adding CommunityJoin, PostJoin instead of joins from GetComments, etc. - Fixes #1122 * Fixing fmt. * Fixing up docs. * Removing translations. * Adding apps / clients to readme. * Fixing main image. * Using new lemmy-isomorphic-ui with better javascript disabled. * Try to fix image uploads in federation test * Revert "Try to fix image uploads in federation test" This reverts commita2ddf2a90b
. * Fix post url federation * Adding some more tests, some still broken. * Don't need gitattributes anymore. * Update local federation test setup * Fixing tests. * Fixing travis build. * Fixing travis build, again. * Changing lemmy-isomorphic-ui to lemmy-ui * Error in travis build again. Co-authored-by: Felix Ableitner <me@nutomic.com>
55 lines
1.5 KiB
Rust
55 lines
1.5 KiB
Rust
use diesel::{result::Error, *};
|
|
use serde::Serialize;
|
|
|
|
table! {
|
|
site_view (id) {
|
|
id -> Int4,
|
|
name -> Varchar,
|
|
description -> Nullable<Text>,
|
|
creator_id -> Int4,
|
|
published -> Timestamp,
|
|
updated -> Nullable<Timestamp>,
|
|
enable_downvotes -> Bool,
|
|
open_registration -> Bool,
|
|
enable_nsfw -> Bool,
|
|
icon -> Nullable<Text>,
|
|
banner -> Nullable<Text>,
|
|
creator_name -> Varchar,
|
|
creator_preferred_username -> Nullable<Varchar>,
|
|
creator_avatar -> Nullable<Text>,
|
|
number_of_users -> BigInt,
|
|
number_of_posts -> BigInt,
|
|
number_of_comments -> BigInt,
|
|
number_of_communities -> BigInt,
|
|
}
|
|
}
|
|
|
|
#[derive(Queryable, Identifiable, PartialEq, Debug, Serialize, QueryableByName, Clone)]
|
|
#[table_name = "site_view"]
|
|
pub struct SiteView {
|
|
pub id: i32,
|
|
pub name: String,
|
|
pub description: Option<String>,
|
|
pub creator_id: i32,
|
|
pub published: chrono::NaiveDateTime,
|
|
pub updated: Option<chrono::NaiveDateTime>,
|
|
pub enable_downvotes: bool,
|
|
pub open_registration: bool,
|
|
pub enable_nsfw: bool,
|
|
pub icon: Option<String>,
|
|
pub banner: Option<String>,
|
|
pub creator_name: String,
|
|
pub creator_preferred_username: Option<String>,
|
|
pub creator_avatar: Option<String>,
|
|
pub number_of_users: i64,
|
|
pub number_of_posts: i64,
|
|
pub number_of_comments: i64,
|
|
pub number_of_communities: i64,
|
|
}
|
|
|
|
impl SiteView {
|
|
pub fn read(conn: &PgConnection) -> Result<Self, Error> {
|
|
use super::site_view::site_view::dsl::*;
|
|
site_view.first::<Self>(conn)
|
|
}
|
|
}
|