mirror of
https://github.com/LemmyNet/lemmy.git
synced 2024-11-05 12:05:01 +00:00
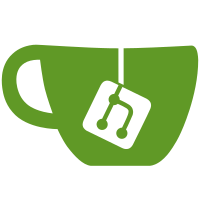
* Make request models derive PartialEq, Eq, and Hash * Fix clippy error --------- Co-authored-by: SleeplessOne1917 <insomnia-void@protonmail.com>
48 lines
1.4 KiB
Rust
48 lines
1.4 KiB
Rust
use lemmy_db_schema::newtypes::CustomEmojiId;
|
|
use lemmy_db_views::structs::CustomEmojiView;
|
|
use serde::{Deserialize, Serialize};
|
|
#[cfg(feature = "full")]
|
|
use ts_rs::TS;
|
|
use url::Url;
|
|
|
|
#[derive(Debug, Serialize, Deserialize, Clone, PartialEq, Eq, Hash)]
|
|
#[cfg_attr(feature = "full", derive(TS))]
|
|
#[cfg_attr(feature = "full", ts(export))]
|
|
/// Create a custom emoji.
|
|
pub struct CreateCustomEmoji {
|
|
pub category: String,
|
|
pub shortcode: String,
|
|
#[cfg_attr(feature = "full", ts(type = "string"))]
|
|
pub image_url: Url,
|
|
pub alt_text: String,
|
|
pub keywords: Vec<String>,
|
|
}
|
|
|
|
#[derive(Debug, Serialize, Deserialize, Clone, PartialEq, Eq, Hash)]
|
|
#[cfg_attr(feature = "full", derive(TS))]
|
|
#[cfg_attr(feature = "full", ts(export))]
|
|
/// Edit a custom emoji.
|
|
pub struct EditCustomEmoji {
|
|
pub id: CustomEmojiId,
|
|
pub category: String,
|
|
#[cfg_attr(feature = "full", ts(type = "string"))]
|
|
pub image_url: Url,
|
|
pub alt_text: String,
|
|
pub keywords: Vec<String>,
|
|
}
|
|
|
|
#[derive(Debug, Serialize, Deserialize, Clone, Copy, Default, PartialEq, Eq, Hash)]
|
|
#[cfg_attr(feature = "full", derive(TS))]
|
|
#[cfg_attr(feature = "full", ts(export))]
|
|
/// Delete a custom emoji.
|
|
pub struct DeleteCustomEmoji {
|
|
pub id: CustomEmojiId,
|
|
}
|
|
|
|
#[derive(Debug, Serialize, Deserialize, Clone)]
|
|
#[cfg_attr(feature = "full", derive(TS))]
|
|
#[cfg_attr(feature = "full", ts(export))]
|
|
/// A response for a custom emoji.
|
|
pub struct CustomEmojiResponse {
|
|
pub custom_emoji: CustomEmojiView,
|
|
}
|