mirror of
https://github.com/LemmyNet/lemmy-ui.git
synced 2024-11-01 18:19:55 +00:00
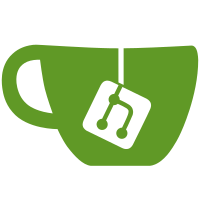
* Beginning work on websocket -> http client conversion. * About 30% done. * half done. * more done. * Almost passing lint. * Passing lint, but untested. * Add back in event listeners. * Fixing some community forms. * Remove webpack cache. * fixing some more. * Fixed ISOwrappers. * A few more fixes. * Refactor utils * Fix instance add/remove buttons * Not catching errors in isoWrapper. * Wrap Http client * Fixing up tagline and ratelimit forms. * Make all http client wrapping be in one place * Reworking some more forms. * Upgrading lemmy-js-client. * Fixing verify email. * Fix linting errors * Upgrading woodpecker node. * Fix comment scrolling rerender bug. * Fixing a few things, commenting out props for now. * v0.18.0-beta.1 * Trying to fix woodpecker, 1. * Trying to fix woodpecker, 2. * Handroll prompt * Add navigation prompt to other pages * Fix prompt navigation bug * Fix prompt bug introduced from last bug fix * Fix PWA bug * Fix isoData not working * Fix search page update url * Fix sharp issue. * v0.18.0-beta.2 * Make create post pre-fetch communities * Fix bug from last commit * Fix issue of posts/comments not being switched when changing select options * Fix unnecessary fetches on home screen * Make circular icon buttons not look stupid * Prevent unnecessary fetches * Make login experience smoother * Add PWA shortcuts * Add related application to PWA * Update translations * Forgot to add post editing. * Fixing site setup. * Deploy script setup. * v0.18.0-beta.4 * Sanitize again. * Adding sanitize json function. * Upping version. * Another sanitize fix. * Upping version. * Prevent search nav item from disappearing when on search page * Allow admin and mod actions on non-local comments. * Fix mobile menu collapse bug * Completely fix prompt component * Fix undefined value checks in use_http_client_2 (#1230) * fix: filter out undefined from posts * fix: emoji initialisation passing undefined * fix: || => ?? to be more explicit * linting --------- Co-authored-by: Alex Maras <alexmaras@gmail.com> * Re-add accidentally removed state * Fix dropdown bug * Use linkEvent where appropriate * Fix navigation warnings. --------- Co-authored-by: Dessalines <tyhou13@gmx.com> Co-authored-by: Alex Maras <dev@alexmaras.com> Co-authored-by: Alex Maras <alexmaras@gmail.com>
162 lines
4.8 KiB
TypeScript
162 lines
4.8 KiB
TypeScript
import { Component, InfernoNode, linkEvent } from "inferno";
|
|
import { T } from "inferno-i18next-dess";
|
|
import {
|
|
ApproveRegistrationApplication,
|
|
RegistrationApplicationView,
|
|
} from "lemmy-js-client";
|
|
import { i18n } from "../../i18next";
|
|
import { mdToHtml, myAuthRequired } from "../../utils";
|
|
import { PersonListing } from "../person/person-listing";
|
|
import { Spinner } from "./icon";
|
|
import { MarkdownTextArea } from "./markdown-textarea";
|
|
import { MomentTime } from "./moment-time";
|
|
|
|
interface RegistrationApplicationProps {
|
|
application: RegistrationApplicationView;
|
|
onApproveApplication(form: ApproveRegistrationApplication): void;
|
|
}
|
|
|
|
interface RegistrationApplicationState {
|
|
denyReason?: string;
|
|
denyExpanded: boolean;
|
|
approveLoading: boolean;
|
|
denyLoading: boolean;
|
|
}
|
|
|
|
export class RegistrationApplication extends Component<
|
|
RegistrationApplicationProps,
|
|
RegistrationApplicationState
|
|
> {
|
|
state: RegistrationApplicationState = {
|
|
denyReason: this.props.application.registration_application.deny_reason,
|
|
denyExpanded: false,
|
|
approveLoading: false,
|
|
denyLoading: false,
|
|
};
|
|
|
|
constructor(props: any, context: any) {
|
|
super(props, context);
|
|
this.handleDenyReasonChange = this.handleDenyReasonChange.bind(this);
|
|
}
|
|
componentWillReceiveProps(
|
|
nextProps: Readonly<
|
|
{ children?: InfernoNode } & RegistrationApplicationProps
|
|
>
|
|
): void {
|
|
if (this.props != nextProps) {
|
|
this.setState({
|
|
denyExpanded: false,
|
|
approveLoading: false,
|
|
denyLoading: false,
|
|
});
|
|
}
|
|
}
|
|
|
|
render() {
|
|
const a = this.props.application;
|
|
const ra = this.props.application.registration_application;
|
|
const accepted = a.creator_local_user.accepted_application;
|
|
|
|
return (
|
|
<div>
|
|
<div>
|
|
{i18n.t("applicant")}: <PersonListing person={a.creator} />
|
|
</div>
|
|
<div>
|
|
{i18n.t("created")}: <MomentTime showAgo published={ra.published} />
|
|
</div>
|
|
<div>{i18n.t("answer")}:</div>
|
|
<div className="md-div" dangerouslySetInnerHTML={mdToHtml(ra.answer)} />
|
|
|
|
{a.admin && (
|
|
<div>
|
|
{accepted ? (
|
|
<T i18nKey="approved_by">
|
|
#
|
|
<PersonListing person={a.admin} />
|
|
</T>
|
|
) : (
|
|
<div>
|
|
<T i18nKey="denied_by">
|
|
#
|
|
<PersonListing person={a.admin} />
|
|
</T>
|
|
{ra.deny_reason && (
|
|
<div>
|
|
{i18n.t("deny_reason")}:{" "}
|
|
<div
|
|
className="md-div d-inline-flex"
|
|
dangerouslySetInnerHTML={mdToHtml(ra.deny_reason)}
|
|
/>
|
|
</div>
|
|
)}
|
|
</div>
|
|
)}
|
|
</div>
|
|
)}
|
|
|
|
{this.state.denyExpanded && (
|
|
<div className="form-group row">
|
|
<label className="col-sm-2 col-form-label">
|
|
{i18n.t("deny_reason")}
|
|
</label>
|
|
<div className="col-sm-10">
|
|
<MarkdownTextArea
|
|
initialContent={this.state.denyReason}
|
|
onContentChange={this.handleDenyReasonChange}
|
|
hideNavigationWarnings
|
|
allLanguages={[]}
|
|
siteLanguages={[]}
|
|
/>
|
|
</div>
|
|
</div>
|
|
)}
|
|
{(!ra.admin_id || (ra.admin_id && !accepted)) && (
|
|
<button
|
|
className="btn btn-secondary mr-2 my-2"
|
|
onClick={linkEvent(this, this.handleApprove)}
|
|
aria-label={i18n.t("approve")}
|
|
>
|
|
{this.state.approveLoading ? <Spinner /> : i18n.t("approve")}
|
|
</button>
|
|
)}
|
|
{(!ra.admin_id || (ra.admin_id && accepted)) && (
|
|
<button
|
|
className="btn btn-secondary mr-2"
|
|
onClick={linkEvent(this, this.handleDeny)}
|
|
aria-label={i18n.t("deny")}
|
|
>
|
|
{this.state.denyLoading ? <Spinner /> : i18n.t("deny")}
|
|
</button>
|
|
)}
|
|
</div>
|
|
);
|
|
}
|
|
|
|
handleApprove(i: RegistrationApplication) {
|
|
i.setState({ denyExpanded: false, approveLoading: true });
|
|
i.props.onApproveApplication({
|
|
id: i.props.application.registration_application.id,
|
|
approve: true,
|
|
auth: myAuthRequired(),
|
|
});
|
|
}
|
|
|
|
handleDeny(i: RegistrationApplication) {
|
|
if (i.state.denyExpanded) {
|
|
i.setState({ denyExpanded: false, denyLoading: true });
|
|
i.props.onApproveApplication({
|
|
id: i.props.application.registration_application.id,
|
|
approve: false,
|
|
deny_reason: i.state.denyReason,
|
|
auth: myAuthRequired(),
|
|
});
|
|
} else {
|
|
i.setState({ denyExpanded: true });
|
|
}
|
|
}
|
|
|
|
handleDenyReasonChange(val: string) {
|
|
this.setState({ denyReason: val });
|
|
}
|
|
}
|